Refactoring in Java
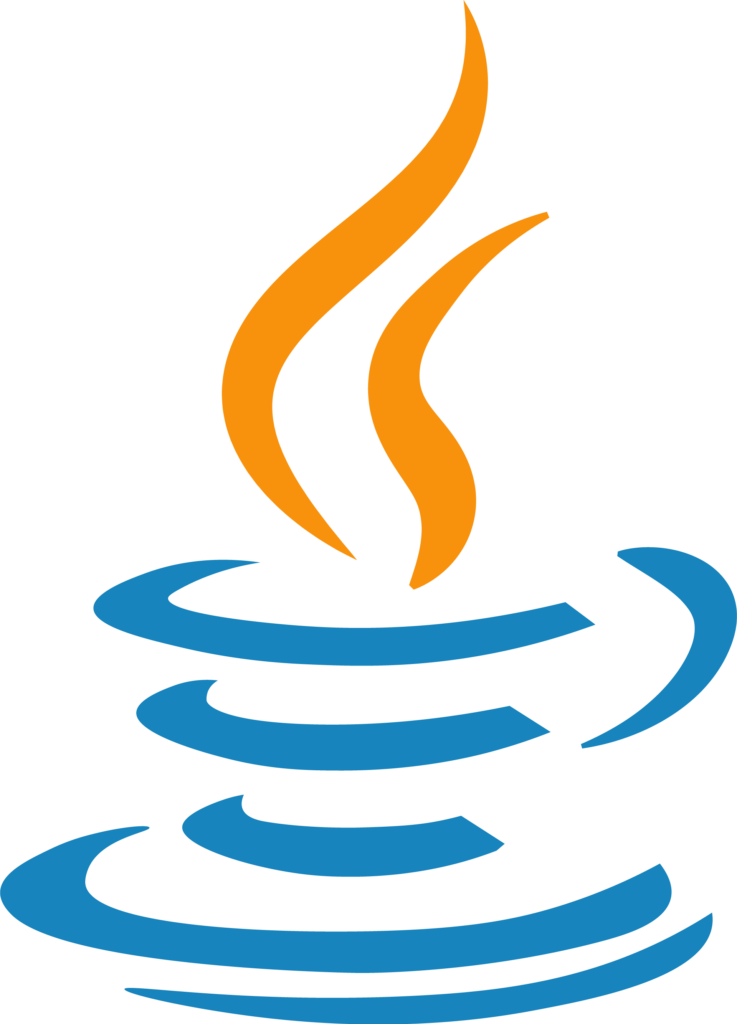
What is refactoring?
Java refactoring is a process of system-wide code changes without affecting the systemโs user interfaces and experiences.
Refactoring Steps
- Create working copies of selected Java files
- Analyze refactoring possibilities
- Apply the refactoring
- Compare working copies with original sources
- Persist wanted refactoring to original sources
Java Refactoring with a Tool
To help developers refactor in Java, we have developed a tool called jSparrow. jSparrow is an IDE extension that aids Java developers in refactoring system-wide Java code with a rule-based approach.
It reviews system-wide code constructs, parameters, strings, temporary files, lambda expressions, if-statements, for-loops, while-loops, forEach-loops, logging statements, and closing statements in Java projects, detects old Java language constructs, and empty variables, and suggests replacements for the identified refactorings. It also allows users to rename fields, remove unused fields, methods, and classes, and replace standard output statements with logger statements.
By installing jSparrow for free to your Eclipse IDE you can view the refactorings on your project directly.
Tool Features
Java refactoring | 118 granular rules, including refactorings for Lambda Logging, Loops, Testing, and more |
Quick-refactorings | 94 quick-fixes which adapt to your code in real-time and provide refactorings |
Three customizable refactorings | Refactor standard output methods to Logger, Remove Unused Code, and Rename Fields to Java Conventions. |
To see which rules are included and other technicalities please read more about jSparrow.
Java Refactoring Examples
Java Refactoring example: Replace Nested Loops with flatMap
One of our most daily visited rules is the โReplace Nested Loops with flatMapโ. This rule finds the nested invocations of Stream::forEach
which are used to iterate over such data structures and replaces them with single invocations of Stream::flatMap
.
Before refactoring:
matrix3.stream().filter(row -> !row.isEmpty()).forEach(row -> {
row.stream().filter(col -> !col.isEmpty()).forEach(col -> {
col.stream().filter(cell -> !cell.isEmpty()).forEach(cell -> {
cell.stream().filter(element -> !element.isEmpty()).map(element -> element.substring(0, 1)).forEach(element -> {
System.out.print(element);
});
});
});
});
After refactoring:
matrix3.stream().filter(row -> !row.isEmpty()).flatMap(row -> row.stream())
.filter(col -> !col.isEmpty())
.flatMap(col -> col.stream())
.filter(cell -> !cell.isEmpty())
.flatMap(cell -> cell.stream())
.filter(element -> !element.isEmpty())
.map(element -> element.substring(0, 1))
.forEach(element -> {
System.out.print(element);
});
Java Refactoring example: Replace Expression Lambda with Method Reference
Another highly visited refactoring rule is the โReplace Expression Lambda with Method Referenceโ. This rule simplifies the expression lambdas by using method reference. Additionally, you can automate this free-forever refactoring rule through our jSparrow Trial.
Before refactoring:
Set<Person> persSet = transferElements(personList, () -> new HashSet<>());
After refactoring:
Set<Person> persSet = transferElements(personList, HashSet::new);
Get Started with jSparrow
The main goal of jSparrow is to help developers automate system-wide Java refactoring tasks. To help developers get started we offer 20 free-forever refactoring rules which you can register for any time.
Rules included to get started:
- Collapse If Statements
- Replace equals() on Enum Constants
- Replace For-Loop with Enhanced-For-Loop
- Replace Expression Lambda with Method Reference
- Split Multiple Variable Declarations
- Use Optional::filter
- Organize Imports
- Use @Override Annotation
- Remove Boxing for String Conversions
- Remove Double Negations
- Remove Unnecessary Semicolons
- Remove Null-Checks Before Instanceof
- Remove Unnecessary Thrown Exceptions on Method Signatures
- Remove toString() on String
- Reorder String Equality Check
- Replace Inefficient Constructors with valueOf()
- Use Try-With-Resource
- Replace Equality Check with isEmpty()
- Use Offset Based String Methods
- Use SecureRandom
jSparrow is a brand of Splendit IT Consulting GmbH